Javascript & the DOM
September 11, 2023
JavaScript and Its Relationship to HTML and CSS
Like HTML and CSS, JavaScript is a language that runs on web browsers. Unlike CSS, which controls the look and design of a web page, and unlike HTML, which describes the content and layout, JavaScript controls the functionality of the website. HTML is like a recipe's ingredient list, containing all the necessary information. CSS is the presentation of the recipe, and JavaScript is like the written instructions on the recipe, controlling the process of making the meal.
Control Flow and Loops
Control flow refers to the order of instructions or steps in a
computer program. If you think of control flow in regular life, it's
like the process of waking up, getting ready for class or work,
commuting there, working or studying, and then coming home, etc. In
programming, loops are a way of repeating a certain operation or set
of instructions concisely. Each weekday for many people is relatively
similar, so if life was like a computer program, you could think of
each day as part of a loop.
There are many kinds of loops in JavaScript. Some examples of common
loops are shown below. In each example, the loops all log each array
element to the console.
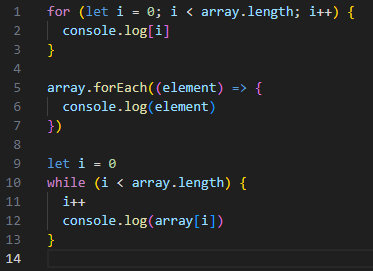
The DOM
The Document Object Model (DOM) is an API interface that represents a
webpage when it loads. It contains lots of information about the page,
enabling different programming languages to interact with it easily.
The DOM follows a tree-like structure, where each node in the tree
represents an object in the document. Many of these objects are often
HTML elements, making using the DOM very convenient for creating
responsive webpages using a language like JavaScript.
In JavaScript, here is an example of how you might access an HTML
element with the id 'box' using the DOM.

Arrays & Objects
Accessing data from arrays and objects in JavaScript is very
different. In an object, because objects contain singular organized
data, it's as simple as specifying what piece of data you want using a
period (.).
Arrays, on the other hand, can be a bit more complicated. Most of the
time, you have to index the array using two square brackets, with the
value inside being the index (position) of the data inside the array.
This creates another problem, as it means you have to know the correct
index value to use to access the correct data inside the array. This
is often solved by programmers by using a loop to cycle through the
array to find the correct piece of data they need from it.

Functions
Functions are handy features in programming that help segment code and make it more reusable. Functions (also called methods) typically cover a certain aspect of the program. This function can then be 'called' anywhere in the scope as many times as needed to execute that code.